MicroPythonの使い心地を知るためにRaspberry Pi Pico Wに安価な1.69インチのTFTディスプレイを接続してNTPで更新した内蔵RTC(リアルタイムクロック)の時刻を表示するプログラムを作りました。
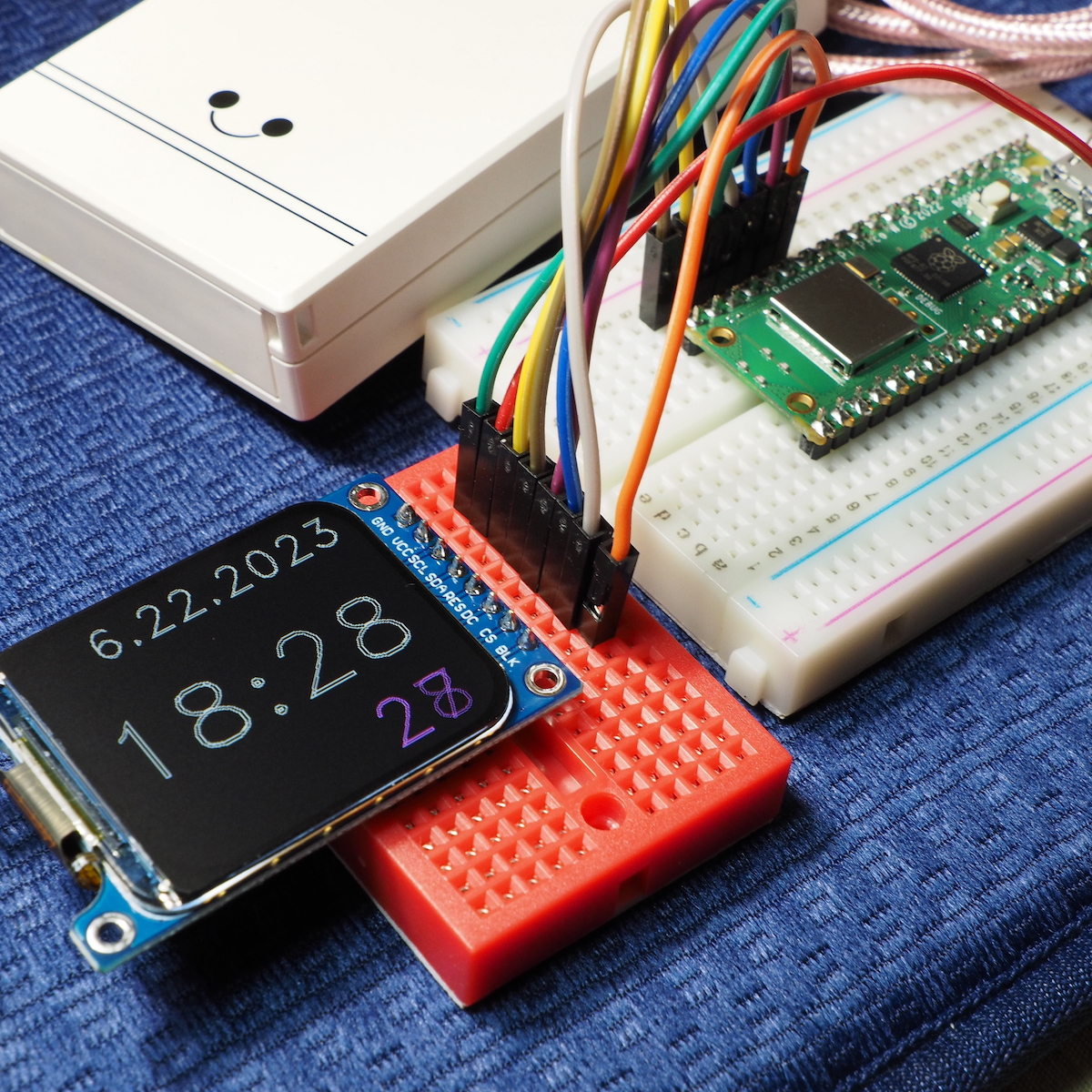
Raspberry Pi Pico W (詳細情報はスイッチサイエンスの商品ページから辿ってください)
1.69インチのTFTディスプレイ (詳細情報はAliexpressの商品ページから辿ってください。400円程度で購入できるSPI通信の240×280画素のフルカラーディスプレイでインターフェースコントローラはst7789を使用していてします。)
配線
<TFTディスプレイ> ——– <Pico W>
GND ——– GND (18pin)
VCC ——– 3V3(OUT) (36pin)
SCL(SCLK) ——– SPI1SCK(19pin)
SDA(MOSI,SDI,DIN) ——– SPI1TX(20pin)
RES(Reset) ——– GP11(15pin)
DC(Data/Command) ——– GP12(16pin)
CS ——– SPI1CSn(17pin)
BLK(Backlight) ——– GP10(14pin)
st7789用ライブラリ
ST7789 Driver for MicroPythonとしてgithubに公開されています。Pico W用MicroPython Firmware (https://github.com/russhughes/st7789_mpy/tree/master/firmware/RP2W/firmware.uf2)をダウンロードして、Pico Wのブートボタンを押しながらPCのUSBポートに接続し、現れたUSBドライブにfirmware.uf2ファイルをドラッグ・ドロップします。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 |
from machine import Pin, SPI import st7789 import utime import network import ntptime_jp SSID = 'yourSSID' PASSWORD = 'YourPassword' BACKLIGHT_PIN = 10 RESET_PIN = 11 DC_PIN = 12 CS_PIN = 13 CLK_PIN = 14 DIN_PIN = 15 # lower left corner import romand as vector_font spi = SPI(1, baudrate=31250000, sck=Pin(CLK_PIN), mosi=Pin(DIN_PIN)) tft = st7789.ST7789(spi, 240, 320, reset=Pin(RESET_PIN, Pin.OUT), cs=Pin(CS_PIN, Pin.OUT), dc=Pin(DC_PIN, Pin.OUT), backlight=Pin(BACKLIGHT_PIN, Pin.OUT), rotation=3) tft.init() sta_if = network.WLAN(network.STA_IF) sta_if.active(True) sta_if.connect(SSID, PASSWORD) while not sta_if.isconnected(): pass ntptime_jp.settime() str_md_c = "" str_hm_c = "" str_s_c = "" while True: time_now = utime.localtime() #print(utime.localtime()) str_md = "{:>2}".format(str(time_now[1])) + "." + "{:>2}".format(str(time_now[2])) + "." + "{:>4}".format(str(time_now[0])) str_hm = "{:>2}".format(str(time_now[3])) + ":" + "{:0>2}".format(str(time_now[4])) str_s = "{:0>2}".format(str(time_now[5])) if str_md != str_md_c : tft.draw(vector_font, str_md_c,50, 30, st7789.color565(0,0,0), 1.4) tft.draw(vector_font, str_md,50, 30, st7789.color565(255,255,255), 1.4) str_md_c = str_md if str_hm != str_hm_c : tft.draw(vector_font, str_hm_c,30, 130, st7789.color565(0,0,0), 2.8) tft.draw(vector_font, str_hm,30, 130, st7789.color565(255,255,255), 2.8) str_hm_c = str_hm if str_s != str_s_c : tft.draw(vector_font, str_s_c,200, 210, st7789.color565(0,0,0), 2.0) tft.draw(vector_font, str_s,200, 210, st7789.color565(255,0,255), 2.0) str_s_c = str_s |
NTP時刻を取得するライブラリは時差を補正することができなかったので、ライブラリ内でJAPAN_TIME = 9*60*60を補正してRTCに書き込むことにしました。ntptime_jp.pyという名前でpico Wに保存しました。(オリジナルはmicropython-lib/micropython/net/ntptime/ntptime.py です。)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 |
import utime try: import usocket as socket except: import socket try: import ustruct as struct except: import struct # The NTP host can be configured at runtime by doing: ntptime.host = 'myhost.org' #host = "pool.ntp.org" host = "ntp.nict.jp" JAPAN_TIME = 9*60*60 # The NTP socket timeout can be configured at runtime by doing: ntptime.timeout = 2 #timeout = 1 timeout = 2 def time(): NTP_QUERY = bytearray(48) NTP_QUERY[0] = 0x1B addr = socket.getaddrinfo(host, 123)[0][-1] s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) try: s.settimeout(timeout) res = s.sendto(NTP_QUERY, addr) msg = s.recv(48) finally: s.close() val = struct.unpack("!I", msg[40:44])[0] EPOCH_YEAR = utime.gmtime(0)[0] if EPOCH_YEAR == 2000: # (date(2000, 1, 1) - date(1900, 1, 1)).days * 24*60*60 NTP_DELTA = 3155673600 elif EPOCH_YEAR == 1970: # (date(1970, 1, 1) - date(1900, 1, 1)).days * 24*60*60 NTP_DELTA = 2208988800 else: raise Exception("Unsupported epoch: {}".format(EPOCH_YEAR)) return val - NTP_DELTA # There's currently no timezone support in MicroPython, and the RTC is set in UTC time. def settime(): t = time() import machine tm = utime.gmtime(t + JAPAN_TIME) machine.RTC().datetime((tm[0], tm[1], tm[2], tm[6] + 1, tm[3], tm[4], tm[5], 0)) |
フォントはst7789_mpy/fonts/vector/romand.pyをPico Wに保存して使いました。